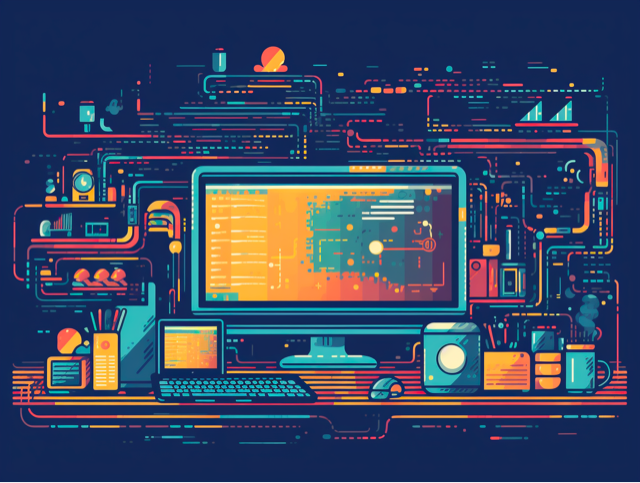
Introduction
Lambda expressions are a powerful feature introduced in C++11 that revolutionized the way we write concise and expressive code. They provide a compact syntax for defining anonymous functions, allowing developers to write inline functions without the need for a named function or function object. In this article, we will explore the capabilities and benefits of C++ lambda expressions and demonstrate how they can enhance your programming experience.1. Concise Syntax for Inline Functions
Lambda expressions provide a concise syntax for defining small, self-contained functions at the point of use. The basic structure of a lambda expression consists of a capture list, parameter list, optional mutable specifier, and a function body. For example: ```cppauto sum = [](int a, int b) { return a + b; };
``` In the above example, we define a lambda expression that takes two integer parameters and returns their sum. The `auto` keyword is used to automatically deduce the lambda expression's type. This concise syntax eliminates the need for writing separate named functions or function objects in many scenarios, improving code readability and maintainability.
2. Capturing Variables from the Surrounding Scope
One of the most powerful features of lambda expressions is the ability to capture variables from the surrounding scope. This allows lambda functions to access and manipulate variables defined outside their own body. The capture list, denoted within square brackets `[ ]`, specifies which variables to capture and how. Lambda expressions support three types of capture:- Value Capture: `[x, y]` captures `x` and `y` by value. The captured variables are read-only within the lambda body.
- Reference Capture: `[&a, &b]` captures `a` and `b` by reference. The captured variables can be modified within the lambda body.
- Mixed Capture: `[x, &y]` captures `x` by value and `y` by reference.
Capture expressions can also be used to capture all variables by value or by reference using `[=]` and `[&]` respectively.
3. Flexibility and Expressiveness
Lambda expressions enable the creation of ad-hoc functions with customized behavior, enhancing the flexibility and expressiveness of C++ code. They can be used in a variety of scenarios, including:- Callback Functions: Lambda expressions can be passed as arguments to functions or algorithms that expect a callable object. This allows for the easy implementation of callbacks, event handlers, or custom sorting functions.
- Algorithm Customization: Many algorithms in the C++ Standard Library, such as `std::sort` or `std::for_each`, accept predicates or comparison functions to customize their behavior. Lambda expressions provide a concise way to define these custom functions directly at the call site.
- Asynchronous Programming: In conjunction with C++11's threading facilities, lambda expressions can be used to define small tasks or work units for parallel execution.
- Stream Operations: Lambda expressions can be used with stream operations in C++ to perform transformations, filtering, or aggregations on collections of data.
4. Improved Readability and Maintainability
Lambda expressions can significantly improve code readability and maintainability by reducing the need for auxiliary functions or function objects. By encapsulating small pieces of logic directly where they are used, lambda expressions make the code more self-contained and easier to understand. They also eliminate the clutter of defining separate functions or objects for simple operations, making the code more concise and focused.Additionally, lambda expressions can help eliminate the verbosity associated with function pointers or traditional function objects, as they provide a more compact and natural syntax for inline functions.
5. Integration with Standard Library Algorithms
C++ lambda expressions seamlessly integratewith the algorithms provided by the C++ Standard Library, enabling powerful and expressive data manipulation. Standard algorithms such as `std::for_each`, `std::transform`, or `std::find_if` can all benefit from lambda expressions as custom predicates or transformations. This integration enhances code clarity, readability, and maintainability by keeping the data processing logic close to the algorithm itself.
Conclusion
Lambda expressions are a valuable addition to the C++ language, providing a concise syntax for defining inline functions with customizable behavior. They enhance code readability, maintainability, and expressiveness by eliminating the need for separate named functions or function objects in many scenarios. Lambda expressions, combined with the flexibility of capturing variables from the surrounding scope, enable powerful and concise code that can be seamlessly integrated with the C++ Standard Library algorithms. Embrace the power of lambda expressions to write more elegant and efficient code in C++.
Article
Be the first comment