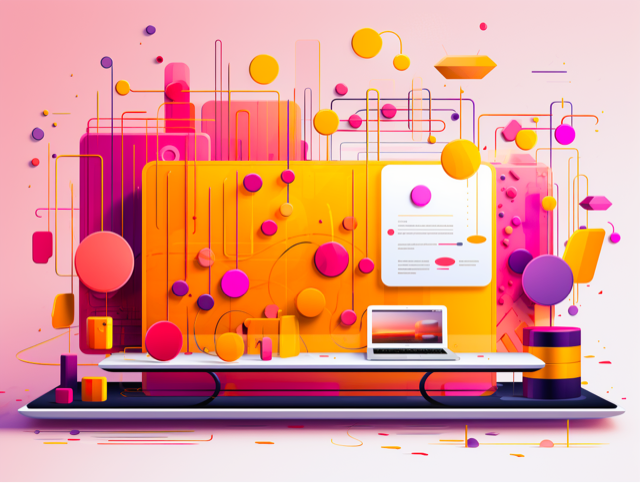
Introduction
Exception handling is a crucial aspect of robust software development, allowing programmers to gracefully handle and recover from unexpected or exceptional situations. C++ provides a comprehensive exception handling mechanism that helps detect, propagate, and handle exceptions. In this article, we will explore the fundamentals of exception handling in C++ and discuss best practices for effective error management.1. Exception Basics
In C++, exceptions are objects that represent exceptional conditions that can occur during program execution. When an exceptional situation arises, such as an error or a violation of a defined condition, an exception is thrown. The program then looks for an appropriate exception handler to catch and handle the exception. The core components of exception handling in C++ are:- `throw`: Used to raise an exception and transfer control to the nearest suitable exception handler.
- `try`: Defines a block of code where exceptions can be thrown and caught. The code within the `try` block is monitored for exceptions.
- `catch`: Defines a block of code that catches and handles specific types of exceptions. It allows the program to gracefully recover from exceptional conditions.
2. Throwing Exceptions
Exceptions are typically thrown using the `throw` keyword followed by an object that represents the exception. The object can be of any type, including built-in types or custom exception classes derived from the `std::exception` base class. For example: ```cppvoid processFile(const std::string& filename) {
if (!openFile(filename)) {
throw std::runtime_error("Failed to open file");
}
// ...
}
``` In the above example, a `std::runtime_error` exception is thrown if the file fails to open.
3. Catching Exceptions
Exceptions are caught and handled using `catch` blocks. A `catch` block specifies the type of exception it can handle and defines the code to be executed when that exception is thrown. For example:```cpp
try {
processFile("data.txt");
} catch (const std::exception& e) {
std::cerr << "Exception caught: " << e.what() << std::endl;
}
```
In the above example, the `catch` block catches exceptions of type `std::exception` (including derived exception classes) and prints an error message.
Multiple `catch` blocks can be used to handle different types of exceptions. The blocks are evaluated in the order they appear, and the first matching block is executed.
4. Exception Safety and Resource Management
Exception safety is a critical aspect of exception handling. It ensures that resources, such as memory or file handles, are properly released even in the presence of exceptions. C++ provides various techniques to achieve exception safety, such as using RAII (Resource Acquisition Is Initialization) and smart pointers.RAII involves tying resource acquisition and release to the lifetime of objects. By encapsulating resources within objects and leveraging destructors, resources are automatically released when the objects go out of scope, regardless of whether an exception occurs.
Smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, provide exception-safe resource management by ensuring that resources are automatically deallocated when the smart pointers go out of scope or are reset.
5. Best Practices for Exception Handling
To effectively handle exceptions in C++, consider the following best practices:- Use specific exception types: Use custom exception classes or standard exception classes derived from `std::exception` to represent specific error conditions. This allows for fine-grained exception handling and clearer code.
- Catch exceptions at an appropriate level: Catch exceptions at a level where meaningful recovery or appropriate error handling can take place. Avoid catching exceptions too early if they cannot be effectively handled at that level.
- Propagate or rethrow exceptions when necessary: If an exception cannot be handled at a particular level, consider propagating it up the call stack or rethrowing it for higher-level exception handlers to handle.
- Provide meaningful error messages: Exception messages should provide sufficient information about the error for debugging and troubleshooting purposes. Include relevant details and context to aid in identifying and resolving the issue.
- Use RAII and smart pointers: Utilize RAII and smart pointers to manage resources, ensuring exception-safe resource allocation and deallocation.
Conclusion
In conclusion, effective exception handling in C++ is essential for writing robust and reliable software. By understanding the basics of exception handling, such as throwing and catching exceptions, developers can gracefully handle exceptional situations and prevent unexpected program termination. By becoming an expert in exception handling strategies and applying them into your C++ development process, you can create software that elegantly manages exceptional circumstances, offers transparent error reporting, and maintains stability and dependability in the face of unforeseen events.
Article
Be the first comment
647 Views
0
0