Posts (36)
Article
Article
Article
Article
Article
Article
Article
Article
Rainbow
2023-06-25
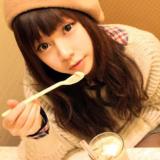
In the vast digital landscape of the World Wide Web, HTML links serve as the magical threads that connect web pages, guiding users from one destination to another with a simple click. Whether it's exploring related content, navigating between sections of a website, or accessing external resources, HTML links are the cornerstone of web navigation. In this article, we will delve into the world of HTML links, their syntax, attributes, and the various ways they can enhance user experiences and facilitate seamless online journeys.
` (anchor) element, with the destination URL specified in the "href" (hypertext reference) attribute. The anchor element typically wraps around the clickable text or image that serves as the link.
Click Here
```
In the example above, "https://www.example.com" is the destination URL, and "Click Here" represents the clickable text displayed to the user. When clicked, the link will direct the user to the specified URL.
Go to Section 2
...
```
In this case, clicking the link "Go to Section 2" will scroll the page to the corresponding section with the ID "section2."
Open in New Window
```
In this case, the link will open the specified URL in a new browser window or tab, while preserving the original page.
Contact Us
```
When clicked, this link will open the user's default email client and populate the "To" field with the specified email address.
Understanding HTML Links
HTML links, also known as hyperlinks or anchors, allow users to navigate between web pages, documents, or different sections within the same page. They are created using the `Basic Link Structure
```htmlLinking to Internal Locations
HTML links can also be used to navigate within the same web page, providing a smooth user experience for content-rich websites. To link to a specific section within a page, you can use an anchor tag with an ID assigned to the target section. Here's an example: ```htmlSection 2
This is the content of section 2.
Opening Links in a New Window or Tab
By default, when a user clicks a link, the destination page replaces the current page in the same browser window. However, you can specify that a link should open in a new window or tab using the "target" attribute. Here's an example: ```htmlLinking to Email Addresses
HTML links can also be used to create email links, allowing users to send messages directly from a web page. To create an email link, use the "mailto" scheme in the "href" attribute, followed by the email address. Here's an example: ```htmlStyling and Enhancing Links
HTML links can be styled and enhanced using CSS to create visually appealing and interactive elements. By applying CSS styles, you can change the link color, underline, hover effects, and more, ensuring that links blend seamlessly with the overall design of your website. Additionally, CSS pseudo-classes like `:hover`, `:visited`, and `:active` can be used to define different styles for links based on their states, such as when the mouse hovers over them or when they have been visited.Accessibility Considerations
When using HTML links, it's crucial to consider accessibility to ensure that all users, including those with disabilities, can navigate the web effectively. Here are a few accessibility best practices for links:1. Use descriptive link text
Instead of generic phrases like "Click Here," use descriptive text that accurately conveys the purpose of the link. This helps users understand where the link will lead them.2. Provide clear visual cues
Ensure that links are visually distinguishable from regular text by using color, underlines, or other styling techniques. Color alone should not be the only indicator of a link.3. Use meaningful link titles
If the link's purpose is not immediately clear from the link text, use the "title" attribute to provide additional context or description.4. Test keyboard accessibility
Ensure that links can be easily navigated using the keyboard alone, without relying solely on mouse interaction.Conclusion
HTML links are the lifelines of the web, connecting web pages and enabling seamless navigation for users. By mastering the syntax, attributes, and best practices of HTML links, you can create engaging and user-friendly websites that facilitate exploration, information retrieval, and smooth online experiences. So, embrace the power of HTML links and let them guide users through the vast digital landscape of the World Wide Web.
Article
Article
Related Users
Elite Article